In this article, we'll discuss the immutability concept and the various implementation of immutability with use cases. According to the MDN definition, An immutable value is one whose content cannot be changed without creating an entirely new value.
Let's understand:
Mutable: These are the objects whose state can be modified after it is created.
Immutable: These are the objects whose state cannot be changed once the object is created.
First of all, there is nothing wrong with mutability or if our code is mutable. But the worry is the misuse of mutability, which can cause a lot of issues including several side effects to our application. Immutability is just a pattern of coding or maintaining the state of an object, making development simple, traceable, and testable. The main goal is, an update should not modify any existing object, but rather create a completely new object.
But why we are so serious about the implementation of immutability? and we already know that javascript (ES6) has const
, by which we can do the same.
const
will provide us with immutable functionality by default, right?
All right, let's discuss, it’s about assigning a variable to a new value, or reassignment. Declared a variable using "let" or "var", it’s possible to reassign variables and values. However, when we try with "const", it'll create issues. Consider the below example one with "let" and another with "const", we are assigning a value "red" into the "color" variable, and whenever we are trying to modify it, it acts differently, which is actually what we are looking for, right?
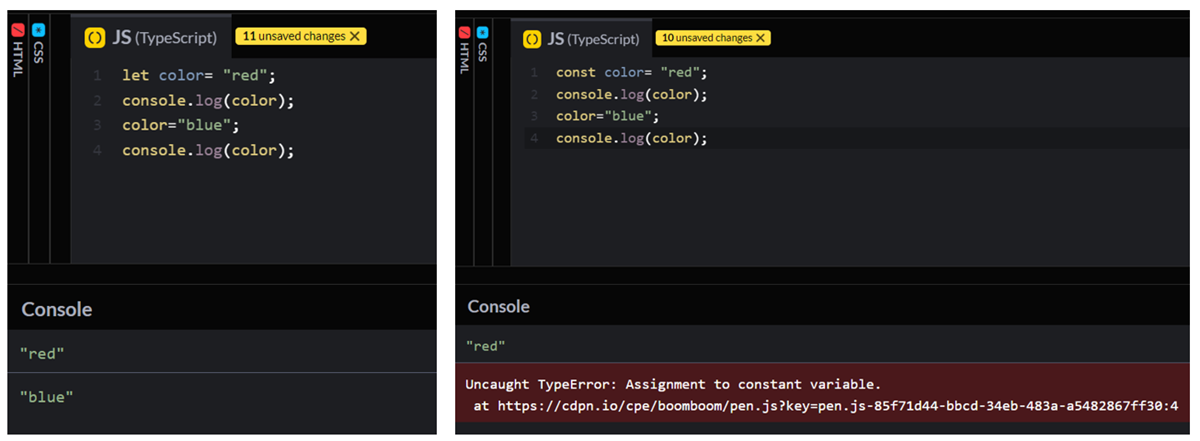
The interesting thing is declaring anything with const doesn’t mean it’s immutable. const means we are not allowed to reassign something. According to MDN,
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned.
Mutable and Immutable Data Types:
In JavaScript:
- All the primitive values are by default immutable. Once a primitive value is declared or created, we cannot change it.
- Objects and arrays are mutable by default. All the properties and elements can be changed without reassigning a new value.
Primitive data types:
There are 7 primitive data types:
- string.
- number.
- bigint.
- boolean.
- undefined.
- symbol.
- null.
Objects and Arrays:
As we discussed above, these are mutable by default. In the below example, we are declaring an object called "color" with some attributes & values with it, such as the “font” attribute having a “red” value. And whenever we are trying to add some other attribute (i.e. "hover") or change any existing attribute’s value (such as the “font” attribute’s value to “green”), it works.
Now take an example of an array we are trying to modify like the above and it also works.