Using Keras to build deep learning model in Cloud Pak for Data 3.5Keras (https://keras.io/) is one of the popular deep learning frameworks used by morden data scientists. IBM Cloud Pak for Data 3.5 preinstalled many open source deep learning frameworks including Keras, Tensorflow and PyTorch. If you want to train deep learning models with Keras in Cloud Pak for Data 3.5, you can easily to get the straight forward development experience in Jupyter notebook of Cloud Pak for Data. This blog provides the instruction and example on how to use Keras training the mnist deep learning model in Cloud Pak for Data 3.5.1. Log into Cloud Pak for Data 3.5 and go into a analytics project, for example keras-mnist-demo2. Add a new notebook with default Python 3.6 runtime
3. Check out the Keras library is loaded in the runtime and load libraries
import warnings
warnings.filterwarnings("ignore")
from sklearn.metrics import classification_report, confusion_matrix
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import Dropout
from keras.utils import np_utils
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
%matplotlib inline
4. Load mnist dataset
mnist_data = mnist.load_data()
(trX, trY), (teX, teY) = mnist_data
print("Number of images for training: {}".format(trX.shape[0]))
print("Number of images used for testing: {}".format(teX.shape[0]))
pix = teX.shape[1]
print("Each image is: {} by {} pixels".format(pix,pix))
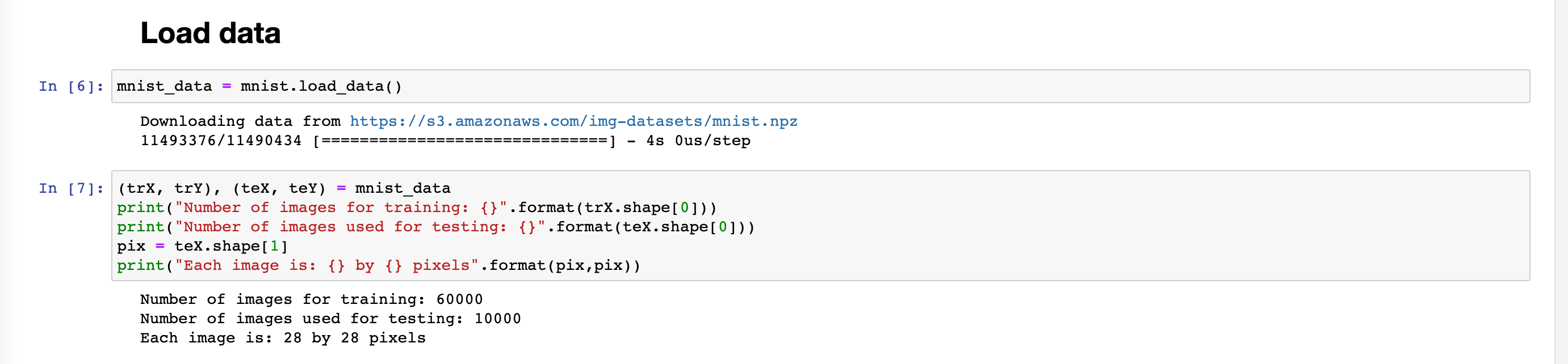
5. Plot some data to see the visual images
plt.subplot(221)
plt.imshow(trX[np.random.randint(60000)], cmap=plt.get_cmap('gray'))
plt.subplot(222)
plt.imshow(trX[np.random.randint(60000)], cmap=plt.get_cmap('gray'))
plt.subplot(223)
plt.imshow(trX[np.random.randint(60000)], cmap=plt.get_cmap('gray'))
plt.subplot(224)
plt.imshow(trX[np.random.randint(60000)], cmap=plt.get_cmap('gray'))
plt.show()
6. Train a simple neural netwrok model with Keras
num_pixels = trX.shape[1] * trX.shape[2]
X_train = trX.reshape(trX.shape[0], num_pixels).astype('float32')
X_test = teX.reshape(teX.shape[0], num_pixels).astype('float32')
X_train = X_train / 255
X_test = X_test / 255
y_train = np_utils.to_categorical(trY)
y_test = np_utils.to_categorical(teY)
num_classes = y_test.shape[1]
model = Sequential()
model.add(Dense(num_pixels, input_dim=num_pixels, kernel_initializer='normal', activation='relu'))
model.add(Dense(num_classes, kernel_initializer='normal', activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10, batch_size=200)
scores = model.evaluate(X_test, y_test, verbose=0)
print(scores)
7. Test the model
def randImg():
i = np.random.choice(np.arange(0, len(teY)), size=(1,))
pred = model.predict(np.atleast_2d(X_test[i]))
image = (X_test[i] * 255).reshape((28, 28)).astype("uint8")
print("Actual digit is {}, model predicted {}".format(teY[i][0], np.argmax(pred[0])))
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
randImg()
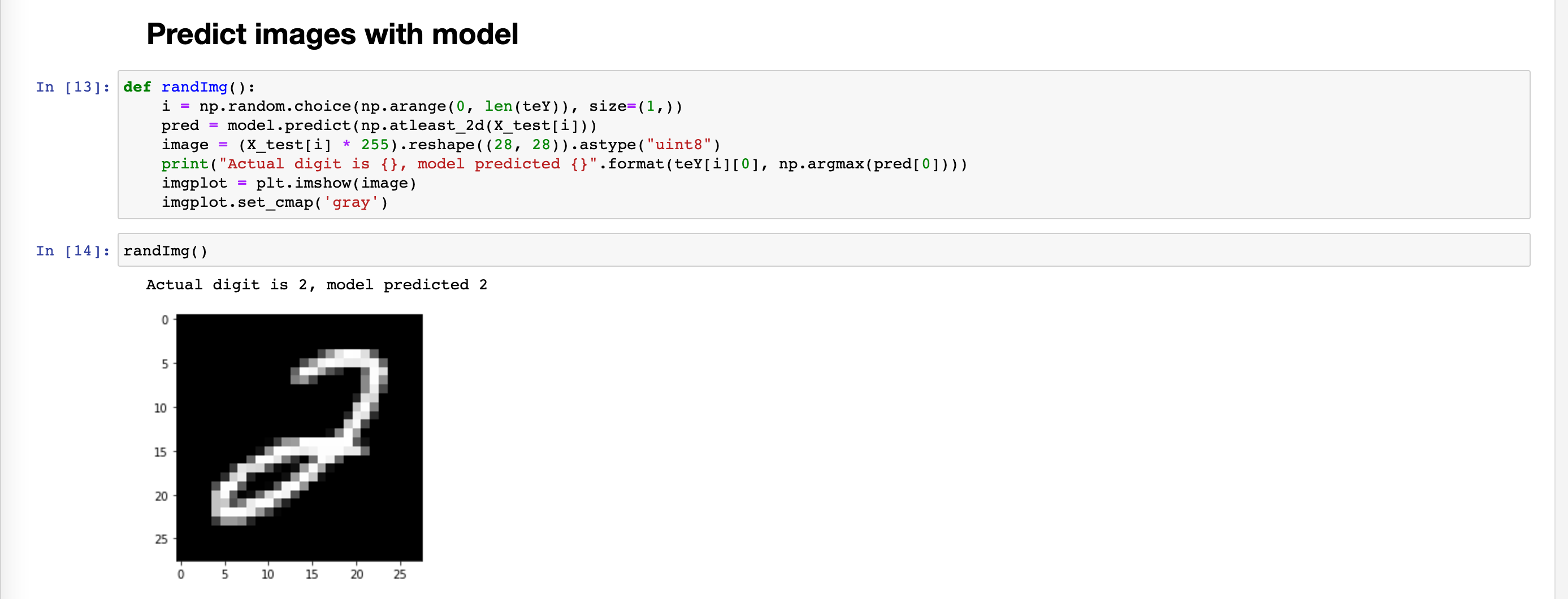
#CloudPakforDataGroup